mirror of
https://tinyplantnews.com/git2/ldaphotos
synced 2025-01-10 18:35:15 +00:00
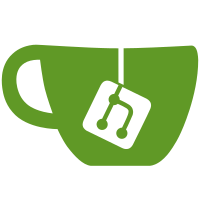
Takes a line of input from stdin, then outputs its SHA256 hash. Verbosity cannot be changed.
71 lines
1.3 KiB
C
71 lines
1.3 KiB
C
#include <openssl/evp.h>
|
|
#include <openssl/err.h>
|
|
#include "log.h"
|
|
|
|
EVP_MD_CTX * md_ctx = NULL;
|
|
EVP_MD * md = NULL;
|
|
|
|
static int hasher_initialized = 0;
|
|
|
|
int init_hasher(void){
|
|
if(hasher_initialized){
|
|
printf("hasher already initialized");
|
|
return 0;
|
|
}
|
|
else{
|
|
md = EVP_MD_fetch(NULL, "SHA256", NULL);
|
|
if(md == NULL){
|
|
ERR_print_errors_fp(stderr);
|
|
return 1;
|
|
}
|
|
md_ctx = EVP_MD_CTX_new();
|
|
/*TODO: see whether EVP_MD_CTX_new() ever fails?*/
|
|
if(md_ctx == NULL){
|
|
ERR_print_errors_fp(stderr);
|
|
return 1;
|
|
}
|
|
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
int deinit_hasher(void){
|
|
if(md != NULL){
|
|
EVP_MD_free(md);
|
|
}
|
|
|
|
if(md_ctx != NULL){
|
|
EVP_MD_CTX_free(md_ctx);
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
int rounduptoblocksize(int a){
|
|
return a;
|
|
}
|
|
|
|
unsigned char * digest(const unsigned char *in, size_t inlen, unsigned int * outlen){
|
|
|
|
if(!EVP_DigestInit_ex(md_ctx, md, NULL)){
|
|
log("Problem encountered while initializing digest");
|
|
ERR_print_errors_fp(stderr);
|
|
return NULL;
|
|
}
|
|
|
|
if(!EVP_DigestUpdate(md_ctx, in, inlen)){
|
|
log("Problem encountered while adding data to digest");
|
|
ERR_print_errors_fp(stderr);
|
|
return NULL;
|
|
}
|
|
|
|
unsigned char *out = OPENSSL_malloc(EVP_MD_get_size(md));
|
|
|
|
if(!EVP_DigestFinal_ex(md_ctx, out, outlen)){
|
|
log("Problem encountered while retrieving digest");
|
|
ERR_print_errors_fp(stderr);
|
|
return NULL;
|
|
}
|
|
|
|
return out;
|
|
}
|